This series of videos demonstrates the step-by-step process of creating and setting up all the essential Azure Services required for constructing a Microservice architecture within the Azure Cloud environment and .Net 6.
Category: Sin categoría
Azure Durable Functions
Durable functions are an extension of Azure Functions that allows Azure Functions to be stateful which mean it allows us to keep track of the context of the application even after the function finished the execution or stopped for some reason allowing you to create workflows easily.
HostedServices .Net Core
Hosted services in .net are classes that implement the IHostedService interface and run background tasks.
These tasks can be started as a part of the host’s lifecycle. That means that they start when the application starts.
The hosted services are useful for performing background tasks such as scheduling, long-running operations, or recurring tasks that need to run independently of the main application.

Factory and Strategy pattern in real case scenario with .Net core 5
Some time ago I got a new requirement. When analyzing the requirement I realized that I would need some design patterns.
When I was first introduced to design patterns I got some trouble understanding them because of the lack of real-life scenarios.
This post contains the usage of the Factory and Strategy pattern in a real-life scenario to create a clean and maintainable code by following the SOLID principles in a real-life scenario.
As a note, it is important to denote that the application follows the CQRS architectural pattern. (You will see the usage of Mediatr library along with some Commands, Queries, and Handlers)

This post is under construction. To read the complete post go to my LinkedIn article
https://www.linkedin.com/pulse/factory-strategy-pattern-real-case-scenario-net-core-5-mata-viejo/
How to handle Validations on CQRS implementation with MediatR .Net core 5
When implementing CQRS using MediatR it is possible to implement pipelines behaviors.
These pipelines allow to catch all commands and queries and gives the ability to execute some logic before and after the Commands and Queries Handlers are executed.
The Validation pipeline behavior
One of the many pipelines that can be added is the validation pipeline behavior which adds logic to validate the properties in the Commands and Queries, resulting in that only valid requests will be passed to the Commands and Queries Handlers.

How to implement Validation Pipeline Behaviour?
The easiest approach is to use FluentValidation. Fluent validation is a library that allows writing easy and complex validations for the Queries and Commands.
The next example shows how to create a Validator.
1.- Implementes AbstractValidator from FluentValidation and add the object to be validated as the parameter.
2.- Write the rules for each one of the parameters of the command or query object.
Implementing Event-Driven in .Net Core 5

The event-driven is an architecture that uses events to communicate changes of states to applications that are interested in different events.
This type of architecture decouples services by using Service buses. This allows the services involved to be scaled, updated, and deployed independently.
This post will explain how to implement an event-driven architecture with MassTransit (a library to configure and send events messages to a Message broker) and RabbitMQ (a message broker that handles events messages).
Continue readingAuto-Generating Angular web client services with Swashbuckle in .Net Core 5
When you are working with Angular and APIs it is very common that models need to be replicated in Angular and then services need to be created in order to call those APIs.
This repetitive job has to be done every time a new model is being created and each time a model is updated in the APIs a manual update has to be done on the Angular model.
Thanks to Swagger, which is the implementation of the Open API specification that comes by default when creating new web APIs in .Net core 5, it is possible to auto-generate client services and models in Angular.
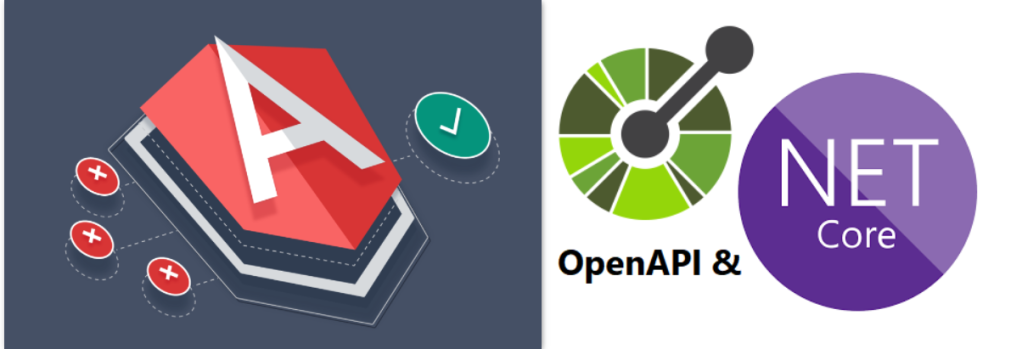
Deploying .Net core microservices with Docker and Kubernetes.

This post will see how to deploy a microservice architecture using Docker and Kubernetes.
This is an extension to the .Net Microservices course where a microservices project is build using .Net Core.
In the course, the author explains how to create a project with .net core using a microservices architecture. The author also shows how to deploy the services using Docker. This extension covers the deployment of the different components in Kubernetes.
Kubernetes helps us to manage containerized applications in different deployment environments. In a microservices environment, it helps to maintain High Availability, Scalability, and Disaster recovery
Let’s first understand what we are going to be deploying in Kubernetes by looking at some diagrams.
GO TO THIS LINK IF YOU ARE NOT ABLE TO SEE THE IMAGES CORRECTLY
https://www.linkedin.com/pulse/deploying-net-core-microservices-docker-kubernetes-david-mata-viejo/
Continue readingHow to intercept, analyze and log secure HTTP requests using .Net core Web API
I was facing a problem where a client app was making an HTTPS request to a server. The server was returning a 400 error.
Usually, these errors are associated with a bad request because the client is sending bad heathers or the body is malformed.
The problem was that the owners of the client app were saying that the application was correct and there was an error in the server.
The server owners were saying that the server was correct and the problem was in the client app.

From 0 to Convolutional Neural Networks
In this post, we are going to understand what a convolutional neural network is and how it works. I’ll be explaining how all the layers are stacked together to form specific architectures. At the end of this post, you will be able to train and test your own convolutional neural network in your own dataset.
I grouped all the information needed to understand what a Convolutional Neural Network is and how it works. This post answers all the questions that I once had when I first started to study CNN and I decided to put them all together.
In the end, I attached the code needed to train your own CNN using Keras. The code is very intuitive and you will easily understand after reading the entire post.
Convolutional neural networks
Convolutional neural networks or just CNNs are a type of deep learning used for image recognition (more correctly “image classification” because it classifies the image into probabilistic classes) and object detection (detecting the coordinates of an object in an image), different architectures are used depending on the aim, for object classification we use Google Inception Net, VGG Net, Let Net, etc. For object detection, we use Single Shot Detectors or YOLO.
